How to Retrieve Emails from Gmail using PHP IMAP
In this article, you will learn how to retrieve emails from Gmail using the PHP IMAP.
Email is the quickest and most important form of communication. We can easily access it from every communication device. It has great importance for small and large organisations. Every day, they deal with hundreds or thousands of emails. They may also demand to read the emails from their applications. Gmail is a free email service provided by Google. Clients can get to Gmail on the web and utilise a third-party program that synchronises email content through POP or IMAP protocols.
PHP provides an IMAP function to access emails from a remote web server to the local end user client. IMAP stands for Internet Message Access Protocol. It is an Internet standard protocol used to access email messages from a mail server. This provides functionality to access the email box from multiple clients and create a web-based email client. There are more than 70 different functions starting with imap_* provided by PHP.
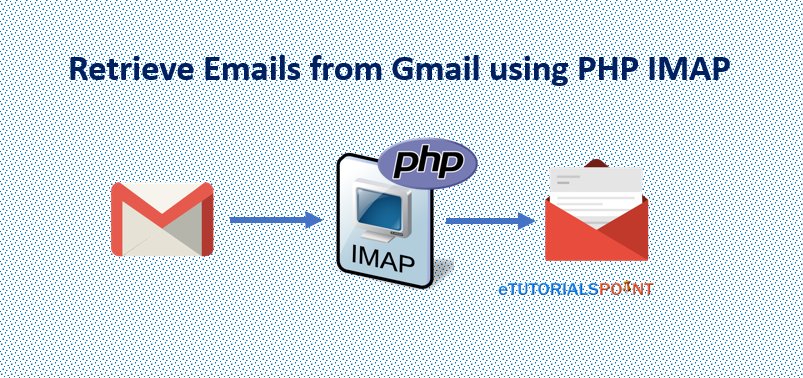
Configuration of IMAP in the PHP server
Before using IMAP in your script, make sure that the IMAP library is installed on your server and enabled in the configuration file. This is by default provided with the WAMP server. You can easily check this in the 'php.ini' file. For this, run the following script and check which ini file is assigned in 'Loaded Configuration File'.
<?php php_info(); ?>
Open the 'php.ini' file and search for the line number to load the imap.
;extension=imap
If the extension is commented like above, remove the semicolon like this and restart the WAMP server to make this effective.
extension=imap
You can check whether IMAP is configured or not using the following code.
if (! function_exists('imap_open')) {
echo "Error: IMAP is not configured.";
exit();
}
It is also necessary to enable IMAP in the Gmail account. For this, go to Gmail Settings->Forwarding and POP/IMAP and enable the IMAP checkbox.
PHP IMAP Functions
These are the IMAP functions with descriptions that we will use in the script to access emails from the Gmail account.
PHP imap_open() function
The most commonly used function is imap_open(). This allows you to create the connection object with the mail server. This requires the following parameters-
imap_open($mailbox, $username, $password)
$mailbox- It consists of the mailbox path of the server.
$username- It contains the username of the mail account.
$password- It contains the password for the mail account.
Example- Connect to GMAIL Account
imap_open('{imap.gmail.com:993/imap/ssl}INBOX','username','password');
The above code opens an IMAP connection to the GMAIL server named imap.gmail.com on port 993. It also passes along with a username and password as the second and third arguments. If you want to connect to the Yahoo account, the IMAP connection code is-
imap_open('{imap.mail.yahoo.com:993/imap/ssl}INBOX','username','password');
You can also connect to the local mail server using the following code.
imap_open('{localhost:993/imap/ssl}INBOX','username','password');
PHP imap_search() function
This function returns messages in an array matching the given search criteria.
imap_search($stream, $criteria)
$stream- It is the IMAP connection object returned by the imap_open() function.
$criteria- These are the search criteria delimited by the spaces. It has some pre-defined constants, like ALL, ANSWERED, BODY, BCC, CC, etc.
PHP imap_fetch_overview() function
It reads an overview of the information in the headers of a given message.
imap_fetch_overview($stream, $sequence)
$stream- It is the IMAP connection object returned by the imap_open() function.
$sequence- It is a message sequence description.
PHP imap_fetchbody() function
It is used to fetch a particular section of the message body.
imap_fetchbody($stream, $number, $section)
$stream- It is the IMAP connection object returned by the imap_open() function.
$number- It is the number of returned messages.
$section- It is the message part number delimited by the period.
PHP IMAP Example
Here is the simple PHP program for retrieving emails from the GMAIL account. In the search criteria, we have passed the keyword 'JOB' to fetch all mails having the keyword 'JOB' in the subject.
<html>
<head>How to Retrieve Emails from Gmail using PHP IMAP</head>
<body>
<h1>Gmail Email Inbox using PHP with IMAP</h1>
<?php
if (! function_exists('imap_open')) {
echo "IMAP is not configured.";
exit();
} else {
?>
<div class="container">
<?php
/* IMAP Connection code with GMAIL IMAP */
$imap_conn = imap_open('{imap.gmail.com:993/imap/ssl}INBOX', 'username', 'password') or die('Cannot connect to Gmail: ' . imap_last_error());
/* SET email subject filter criteria */
$inbox = imap_search($imap_conn, 'SUBJECT "JOB"');
if (! empty($inbox)) {
?>
<table class="table table-striped">
<?php
foreach ($inbox as $email) {
// Get email header information
$overview = imap_fetch_overview($imap_conn, $email, 0);
// Get email body
$message = imap_fetchbody($imap_conn, $email, '2');
$date = date("d F, Y", strtotime($overview[0]->date));
?>
<tr>
<td>
<?php echo $overview[0]->from; ?>
</td>
<td>
<?php echo $overview[0]->subject; ?> - <?php echo $message; ?>
</td>
<td>
<?php echo $date; ?>
</td>
</tr>
<?php
}
?>
</table>
<?php
}
// Close imap connection
imap_close($imap_conn);
}
?>
</div>
</body>
</html>
Related Articles
PHP Countdown TimerPerfect number program in PHP
Check if value exists in array PHP
PHP reverse a string without predefined function
PHP random quote generator
PHP convert string into an array
PHP remove HTML and PHP tags from string
Import Excel File into MySQL using PHP
PHP array length
How to send emojis in email subject and body using PHP
Sending form data to an email using PHP
Simple way to send SMTP mail using Node.js PHP code to send email using SMTP
JavaScript display PDF in the browser using Ajax call
jQuery loop over JSON result after AJAX Success
Simple star rating system using PHP, jQuery and Ajax
jQuery File upload progress bar with file size validation
Print section of page using javascript
Submit a form data without page refresh using PHP, Ajax and Javascript