How to store emoji characters in MySQL using PHP
In this article, you will learn how to store a user's comment with emoji characters in the MySQL database using the PHP programming language.
Emojis are used to express your feelings, emotions, work, or something in just a single icon. It was a great achievement of Emoji when it was standardised in Unicode. The Unicode 12.0 contains emoji using 1,311 characters. With the popularity of emoji, most programming languages have introduced syntax to construct full unicode for emoji and international characters. Now, there are several third party plugins available in JavaScript to add these emoji pickers in input fields.
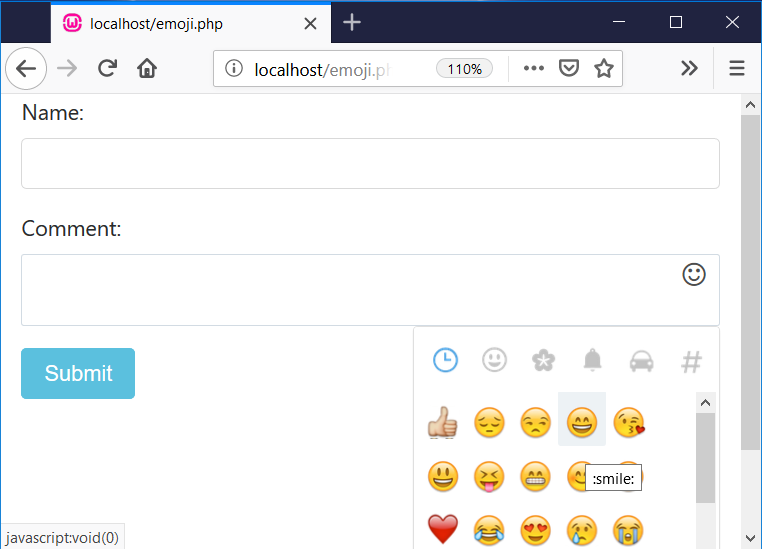
These are the following step-by-step instructions to add the emoji picker plugin to the HTML input box and store it in the database.
For this, we have created a MySQL TABLE 'userscomment'. You can use your existing database if you have one.
CREATE TABLE IF NOT EXISTS `userscomment`
(
`uid` int(11) NOT NULL AUTO_INCREMENT PRIMARY KEY,
`name` varchar(100) DEFAULT NULL,
`comment` varchar(150) CHARACTER SET utf8mb4 COLLATE utf8mb4_bin NOT NULL
)ENGINE=InnoDB AUTO_INCREMENT=90 DEFAULT CHARSET=utf8mb4;
To store the unicode in MySQL, the right CHARSET value is important. In the above CREATE statement, we have set the comment fields CHARACTER and COLLATE as.
CHARACTER SET utf8mb4 COLLATE utf8mb4_bin
And, also set the TABLE DEFAULT CHARSET 'utf8mb4'.
Now, to insert emoji in comment input, we need an emoji picker library. There are several third-party jQuery emoji picker libraries available. In this example, we have taken the emoji-picker library from github. You can download this from here-
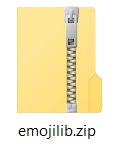
To invoke the emoji picker in the input field, add (data-emojiable="true" data-emoji-input="unicode") attributes in the input element and enabled the picker using js code.
$(function() {
window.emojiPicker = new EmojiPicker({
emojiable_selector: '[data-emojiable=true]',
assetsPath: 'emojilib/img/',
popupButtonClasses: 'fa fa-smile-o'
});
window.emojiPicker.discover();
});
index.php
Here is the main PHP file, 'index.php' that we will call in the browser. We have created a form that contains one input field for name and a textarea to enter a comment with emojis. In the head section of HTML, we have included the Bootstrap CSS and emoji picker CSS files. Make sure the library path is correct. All the jQuery files of the library are added at the end of the body section.
<?php include('storedata.php'); ?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.6/css/bootstrap.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.4.0/css/font-awesome.min.css">
<link href="emojilib/css/emoji.css" rel="stylesheet">
</head>
<body onload="getData()">
<form action="#" method="post">
<div class="container">
<div class="form-group">
<label for="usr">Name:
<input type="text" class="form-control" id="usr" name="name">
</div>
<div class="form-group">
<label for="comment">Comment:</label>
<p class="lead emoji-picker-container">
<textarea class="form-control" rows="1" data-emojiable="true" data-emoji-input="unicode" name="comment"></textarea>
</p>
</div>
<div class="form-group">
<input type="submit" class="btn btn-info" value="Submit">
</div>
</div>
</form>
<div class="form-group">
<div id="comments"></div>
</div>
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script src="emojilib/js/config.js"></script>
<script src="emojilib/js/util.js"></script>
<script src="emojilib/js/jquery.emojiarea.js"></script>
<script src="emojilib/js/emoji-picker.js"></script>
<script>
$(function() {
window.emojiPicker = new EmojiPicker({
emojiable_selector: '[data-emojiable=true]',
assetsPath: 'emojilib/img/',
popupButtonClasses: 'fa fa-smile-o'
});
window.emojiPicker.discover();
});
function getData(uid) {
$.ajax({
url: 'handler.php?uid='+uid,
success: function(html) {
var ajaxDisplay = document.getElementById('comments');
ajaxDisplay.innerHTML = html;
}
});
}
</script>
</body>
</html>
We have added a js function getData() that calls the ajax method to dynamically fetch the entered comment of the user from the database and load it within the div element having ID 'comments'.
In the 'index.php' file, we have added a PHP file 'storedata.php'. This file contains code to insert data into 'usercomment' TABLE using MySQLi. Make sure to replace the 'hostname', 'username', 'password' and 'database' with your database credentials and name.
storedata.php
<?php
$username = $_POST['name'];
$comment = $_POST['comment'];
$conn = mysqli_connect('hostname', 'username', 'password', 'database');
//Check for connection error
if($conn->connect_error){
die("Error in DB connection: ".$conn->connect_errno." : ".$conn->connect_error);
}
if($_POST['name'] && $_POST['comment']){
$insert = 'INSERT INTO `userscomment` (`uid`, `name`, `comment`)
VALUES (`uid`, "'.$username.'", "'.$comment.'")';
if(mysqli_query($conn, $insert)){
$uid = mysqli_insert_id($conn);
}
}
?>
Here, we store the last inserted ID in the '$uid' variable. This is passed as a parameter to the 'getData()' javascript function. This function calls 'handler.php' file using ajax and shows the entered user's name and comment with emojis.
handler.php
<?php
if($_GET['uid']) {
$uid = $_GET['uid'];
$conn = mysqli_connect('hostname', 'username', 'password', 'database');
//Check for connection error
if($conn->connect_error){
die("Error in DB connection: ".$conn->connect_errno." : ".$conn->connect_error);
}
$select = "SELECT * FROM `userscomment` WHERE uid = '$uid' ";
$result = $conn->query($select);
if($result->num_rows > 0){
while($row = $result->fetch_object()){
echo '<strong>Name: </strong>'.$row->name.'<br/>'.
'<strong>Comment: </strong>'.$row->comment.'<br/>';
}
}
}
?>
Related Articles
SQL Injection Prevention TechniquesPHP code to send email using SMTP
PHP code to send SMS to mobile from website
PHP Sending HTML form data to an Email
Fibonacci Series Program in PHP
PHP remove last character from string
How to display PDF file in PHP from database
Submit a form data without page refresh using PHP, Ajax and Javascript
PHP Server Side Form Validation
How to add google reCAPTCHA v2 in registration form using PHP
Complete HTML Form Validation in PHP
Simple star rating system using PHP, jQuery and Ajax
jQuery File upload progress bar with file size validation
How to read CSV file in PHP and store in MySQL
Generating word documents with PHP
PHP SplFileObject Examples
How to Upload a File in PHP
Simple PHP email form
Password reset system in PHP
HTTP authentication with PHP
PHP file cache library