Check if two strings are anagrams Python
In this post, you will learn how to write Python program to check if two strings are anagrams or not.
An anagram of a string is another string that contains the same characters, only the order of the characters can be different. For example, triangle and integral are anagram strings. Both strings contain the same set of characters.
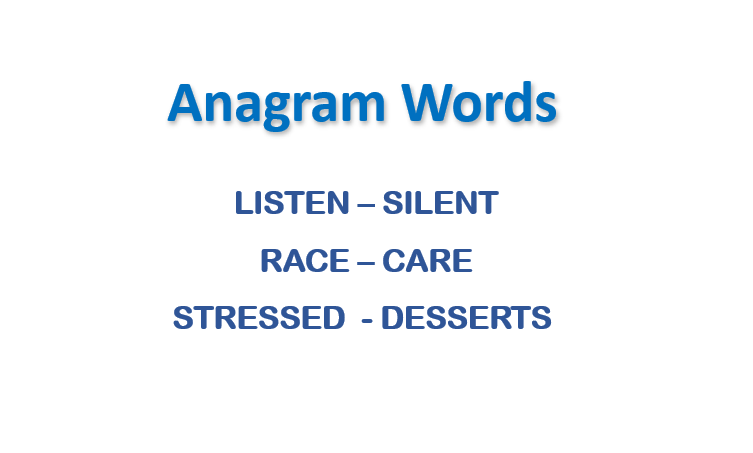
So, if we need to check if two strings are an anagram or not, we will have to check if both strings contain the same characters or not.
Python program for anagram strings
In the given Python program, we have taken two input strings from the user and stored them in two separate variables. Next, we have sorted both strings alphabetically and compared both strings to see if they are equal or not. If equal, they should be an anagram. Otherwise not.
def checkAnagram(str1,str2):
return sorted(str1) == sorted(str2)
# Get string inputs
str1 = input("Enter the string 1 : ")
str2 = input("Enter the string 2 : ")
# convert both the strings into lowercase
str1 = str1.lower()
str2 = str2.lower()
if checkAnagram(str1,str2):
print("Strings are anagram")
else:
print("Strings are not anagram")
Output1:
Enter the string 1 : Race
Enter the string 2 : Care
Strings are anagram
Output2:
Enter the string 1 : Silent
Enter the string 2 : listen
Strings are anagram

Python check if two strings are anagrams using sorted() function
Python provides a predefined function sorted() that does not modify the original string, but returns a sorted string. Here, we check anagram strings using sorted() function.
def check(str1, str2):
# the sorted strings are checked
if(sorted(str1)== sorted(str2)):
print("The strings are anagrams.")
else:
print("The strings aren't anagrams.")
# driver code
str1 ="care"
str2 ="race"
check(str1, str2)
Python check if two strings are anagrams using counter() function
Python Counter is a holder that will hold the count of each of the elements present in the container. The counter is a sub-class accessible inside the dictionary class. It works by putting each character as a key and counting them as dictionary values. Here, we use the counter() function to check if two strings are anagrams.
from collections import Counter
# function to check if two strings are
# anagram or not
def check_anagrams(str1, str2):
if(Counter(str1) == Counter(str2)):
print("The strings are anagrams.")
else:
print("The strings aren't anagrams.")
# driver code
x = "listen"
y = "silent"
check_anagrams(x, y)
Output of the above code:
The strings are anagrams.
Related Articles
Convert a string to a float in Python
Casefold in Python
isalpha Python
Python split multiple delimiters
Replace multiple characters Python
Python multiline string
Alphabet pattern programs in Python
Python remove punctuation from string
Remove character from string Python
Count vowels in a string python
Count occurrences of each character in string Python
Convert string to list Python
Python split multiple delimiters
Python program to reverse a string
Permutation of string in Python
Remove spaces from string Python
splitlines in python
Python program to sort words in alphabetical order
Python split string by comma
Remove character from string Python
Check if two strings are anagrams Python