Python convert XML to JSON
In this article, you will learn how to convert from XML to JSON using the Python programming language. Python has a bunch of supportive libraries and packages that limit the usage of code in our regular daily life. This nature of Python makes it a hot pick among a large portion of the development community, particularly for data scientists.
XML plays an important role in a developer's life. It provides a structured way to mark up data arranged in a tree-like hierarchy. It is also known as document representation language. As it is rendered easily in many web applications, it becomes a good choice in web services and data exchange. As it reduces complexity and data can be read by different incompatible applications, it becomes a good choice in web services and data exchange. In general, XML can be somewhat heavier. You're sending more information, which implies you need more transfer speed, more extra room, and more run time.
Both XML and JSON are used for transferring data between a client and a server. But, JSON has been displaced by XML in many basic situations. As, JSON is more lightweight and faster to parse than XML. A JSON object contains data in the form of a key/value pair. The keys are strings, and the values are the JSON types. They are separated by a colon. Data in JSON can be easily accessible as objects. It provides an automated way to easily serialize and deserialize JavaScript.
In this article, you will learn to convert data from XML to JSON using the Python programming language.
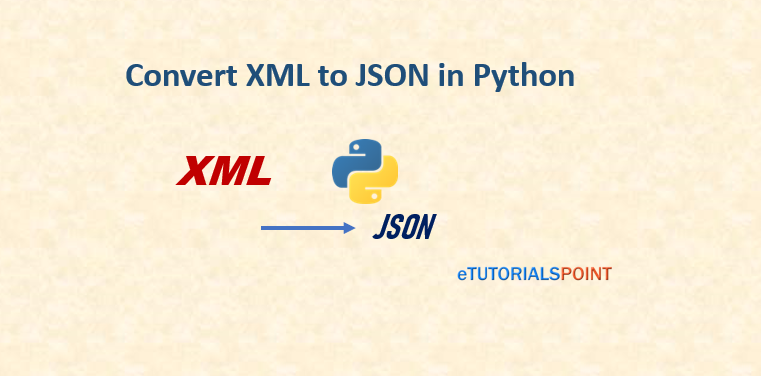
Python converting XML data to JSON
Suppose we have the following XML that contains student records-
<?xml version="1.0" encoding="UTF-8" ?>
<root>
<stu1 type="dict">
<name type="str">Priska</name>
<class type="str">3A</class>
<year type="int">2014</year>
</stu1>
<stu2 type="dict">
<name type="str">Somiya</name>
<class type="str">2A</class>
<year type="int">2016</year>
</stu2>
<stu3 type="dict">
<name type="str">Adam</name>
<class type="str">4C</class>
<year type="int">2012</year>
</stu3>
</root>
Install xmltodict module
To generate XML, first we need to install the xmltodict module using the following command.
pip3 install xmltodict
After the successful installation, the above command returns a message that looks something like this-
Installing collected packages: xmltodict
Successfully installed xmltodict-0.12.0
Here is the complete code to convert XML to JSON using Python. There are several libraries and methods available to convert from XML, but we are using a simple Python module 'xmltodict' to convert data from XML.
In the given code, we have imported three modules. The xmltodict module is used to convert data from xml to dictionary. The xmltodict.parse() method parses the given XML input and converts it into a dictionary. The pprint module provides a definition of PrettyPrinter class that represents the data in a formatted way. The indent parameter defines indentation added on each recursive level. The default value is 1. The use of the json module to pretty print the JSON data. The json.dumps() method takes a JSON object and returns a string in JSON format.
import xmltodict
import pprint
import json
my_xml = """<?xml version="1.0" encoding="UTF-8" ?>
<root>
<stu1 type="dict">
<name type="str">Priska</name>
<class type="str">3A</class>
<year type="int">2014</year>
</stu1>
<stu2 type="dict">
<name type="str">Somiya</name>
<class type="str">2A</class>
<year type="int">2016</year>
</stu2>
<stu3 type="dict">
<name type="str">Adam</name>
<class type="str">4C</class>
<year type="int">2012</year>
</stu3>
</root>
"""
my_dict = xmltodict.parse(my_xml)
pp = pprint.PrettyPrinter(indent=4)
pp.pprint(json.dumps(my_dict))
The above code returns the following output -
('{"root": {"stu1": {"@type": "dict", "name": {"@type": "str", "#text": '
'"Priska"}, "class": {"@type": "str", "#text": "3A"}, "year": {"@type": '
'"int", "#text": "2014"}}, "stu2": {"@type": "dict", "name": {"@type": "str", '
'"#text": "Somiya"}, "class": {"@type": "str", "#text": "2A"}, "year": '
'{"@type": "int", "#text": "2016"}}, "stu3": {"@type": "dict", "name": '
'{"@type": "str", "#text": "Adam"}, "class": {"@type": "str", "#text": "4C"}, '
'"year": {"@type": "int", "#text": "2012"}}}}')
Python convert XML file to JSON file
In the given code, we have converted an XML file to a JSON file. First, we have imported the necessary modules- xmltodict, pprint, and json. Then, we open the input XML file and read the data in the form of a Python dictionary. Next, we got the JSON formatted string with the help of json.dumps and wrote the data to a JSON file.
import xmltodict
import pprint
import json
# open the input xml file and read data
with open("employee.xml") as xml_file:
my_dict = xmltodict.parse(xml_file.read())
xml_file.close()
json_data = json.dumps(my_dict)
# write the json data to output
with open("employee.json", "w") as json_file:
json_file.write(json_data)
json_file.close()
pp = pprint.PrettyPrinter(indent=4)
pp.pprint(json_data)
The above code generates the given JSON file in output-
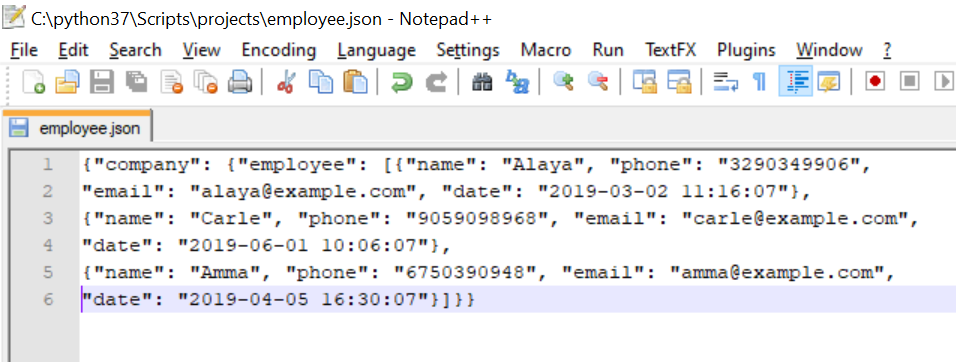
Related Articles
Eye Detection Program in Python OpenCVInstall NLTK for Python on Windows 64 bit
Find the stop words in nltk Python
How to read xml file in Python
Python Spell Checker Program
Python remove punctuation from string
How to convert Excel to CSV Python Pandas
How to read data from excel file using Python Pandas
How to read data from excel file in Python
Python read JSON from URL requests
CRUD operations in Python using MYSQL Connector
Fibonacci Series Program in Python
Python File Handler - Create, Read, Write, Access, Lock File
Python convert xml to dict
Python convert dict to xml
How to generate QR Code in Python using PyQRCode
Python programs to check Palindrome strings and numbers