JavaScript display PDF in the browser using Ajax call
In this article, you will learn how to display PDF in the browser from Ajax response using JavaScript.
Ajax provides the ability to dynamically change portions of a web page without reloading the complete web page. Some portions of a web page are static, like the header, footer, and menus, that need to be reloaded when the user interacts with the page. The reloading of the page takes extra user time. You have experienced that when you click on some request, that page may hang because a lot of information is trying to load at once.
Ajax provides a facility to dynamically update some portions of a web page without disturbing or reloading the other portions of the web page. For this, we just have to develop a container to display output from a program hosted on a web server. Here is the basic example of how to display PDF files within the web page content using AJAX call.
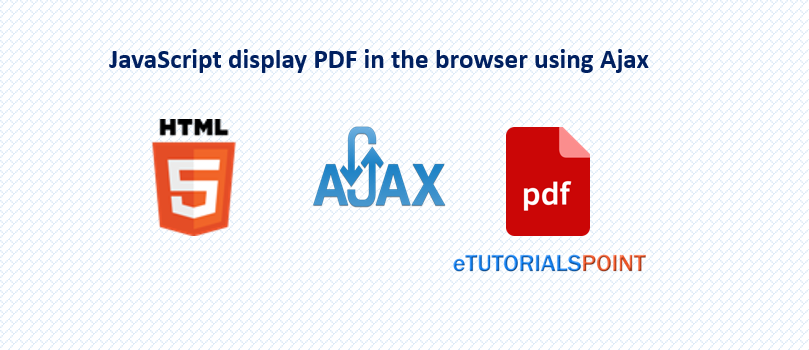
index.html
First, we have created a main HTML file that we will call in the browser. This file contains basic HTML code. We have added a button 'Click Here'. When the user clicks on this, it calls a JavaScript function called 'ajaxRequest()'. In the header section of this page, we have included one style sheet file 'style.css' and one JavaScript file 'ajax.js'-
<html>
<head>
<title>Javascript Ajax Demo</title>
<meta charset="utf-8">
<link href="style.css" rel="stylesheet">
<script type='text/javascript' src='ajax.js'></script>
</head>
<body>
<div id="wrapper">
<div id="header">
<h1>Welcome to eTutorialsPoint</h1>
</div>
<div id="content">
<h2>Information</h2>
<p>You can view the information in detail on click the below button-</p>
<br/>
<input type='button' onclick='ajaxRequest();' value='Click Here'/>
<br/>
<div id='getAjaxResponse'>
When you click the above button, this section changes and load PDF file.
</div>
</div>
<div id="footer">
Copyright © 2019 etutorialspoint.com
</div>
</div>
</body>
</html>
style.css
Here is the stylesheet 'style.css' to enhance the user interface, that we have included in the above 'index.html' file.
body { background-color: #CCCCCC ;
font-family: Arial, Verdana, sans-serif; }
h1 { margin: 10px; }
h2 { color: #000000;
font-family: Arial, sans-serif; }
#wrapper { margin: 0 auto;
width: 85%;
min-width: 800px;
background-color: #0093D1;
color: #000066; }
#header { background-color: #0093D1;
color: #00005D; }
#content { background-color: #ffffff;
color: #000000;
padding: 10px 20px;
overflow: auto; }
#footer { font-size: 80%;
text-align: center;
padding: 5px;
background-color: #0093D1;
color: #ffffff;
clear: both;}
ajax.js
Here is the JavaScript 'ajax.js' file, that we have called on 'index.html' page.
function getXMLHttp()
{
var xmlHttp;
try
{
xmlHttp = new XMLHttpRequest();
}
catch(e)
{
//Internet Explorer is different than the others
try
{
xmlHttp = new ActiveXObject("Msxml2.XMLHTTP");
}
catch(e)
{
try
{
xmlHttp = new ActiveXObject("Microsoft.XMLHTTP");
}
catch(e)
{
alert("You have Old Browser! Please update to use AJAX call.")
return false;
}
}
}
return xmlHttp;
}
function ajaxRequest()
{
var xmlHttp = getXMLHttp();
xmlHttp.onreadystatechange = function()
{
if(xmlHttp.readyState == 4)
{
handleResponse(xmlHttp.responseText);
}
}
xmlHttp.open("GET", "doc.html", true);
xmlHttp.send(null);
}
function handleResponse(response)
{
document.getElementById('getAjaxResponse').innerHTML = response;
}
doc.html
Here is the HTML file that has an embedded PDF file using the iframe element. The whole of the HTML page content will be placed within the div id 'getAjaxResponse' on Ajax call.
<h1>Here is the detail information in PDF</h1>
<iframe src="document/etp.pdf" width="90%" height="500px">
</iframe>
Related Articles
Remove duplicates from array JavaScriptHow to reverse a number in JavaScript
Select/deselect all checkboxes using JavaScript
Print specific part of a web page in JavaScript
JavaScript speech recognition example
Submit a form data without page refresh using PHP, Ajax and Javascript
How to convert associative array to XML in PHP
Get Visitor Information by IP Address in PHP
Getting Document of Remote Address
Get Visitor's location and TimeZone
Get current visitor's location using HTML5 Geolocation API and PHP
JavaScript display PDF in the browser using Ajax call
How to generate PDF file using mPDF in PHP
Export data from MySQL table to CSV file using php
How to display PDF file in PHP from database
Polling System using PHP, MySQL and Ajax
How to read CSV file in PHP and store in MySQL
PHP File Upload MIME Type Validation with Error Handler
File Upload Validation in PHP