PHP File Upload MIME Type Validation with Error Handling
In this post, you will learn how to get the file mime type validation using the PHP programming language.
While uploading a file, there may be a need to validate the uploaded file type, file size, existence of the uploaded file, and so on. In this article, you will learn to develop a PHP file upload script that allows only limited file extensions and MIME file type validation with different error handlers. PHP provides HTTP File Upload variables $_FILES, which is an associative array containing uploaded items via the HTTP POST method.
For example, you are developing an application that contains a file upload form. You only need to upload PDF, DOC, and DOCX files and want to provide validation on the server side. Let's learn how to achieve this.
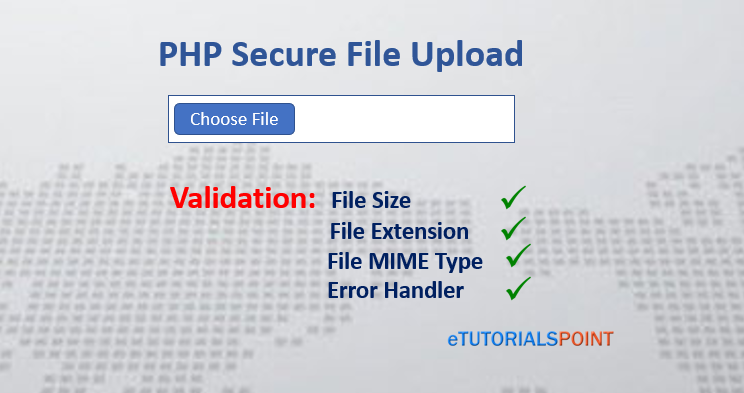
File Upload Form
First, create a main PHP file 'index.php' that contains a file upload form. This is the main file that we will call in the browser.
<html>
<head>
<title>File Upload Validation in PHP</title>
</head>
<body>
<form action='#' method="post" enctype="multipart/form-data">
<input type="file" name="file" />
<input type="Submit" value="Submit" />
</form>
</body>
</html>
Configure File Size Limit and Upload Directory
At the top of the 'index.php' page, we take two constants, UPLOAD_DIR and MAXSIZE, using PHP and define the upload directory and maximum allowed file size, respectively.
define('UPLOAD_DIR', '/var/uploaded_files/');
define('MAXSIZE', 7340032); // allow max 7 MB
Configure Allowed File Type
Next, we define all allowed file extensions in an array $ALLOWED_FILEEXT and all allowed MIME types in an array $ALLOWED_MIME.
$ALLOWED_FILEEXT = array('pdf', 'doc', 'docx' );
$ALLOWED_MIME = array('application/pdf',
'application/msword',
'application/vnd.openxmlformats-officedocument.wordprocessingml.document');
Next, create a function allowedfile() that accepts a temporary file name and destination path as parameters. We get the uploaded file extension using the PHP predefined function pathinfo() and the mime type of the uploaded file using the mime_content_type() function. The allowedfile() function returns TRUE if the uploaded file's file extension and MIME type are both allowed.
function allowedfile($tempfile, $destpath) {
global $ALLOWED_FILEEXT, $ALLOWED_MIME;
$file_ext = pathinfo($destpath, PATHINFO_EXTENSION);
$file_mime = mime_content_type($tempfile);
$valid_extn = in_array($file_ext, $ALLOWED_FILEEXT);
$valid_mime = in_array($file_mime, $ALLOWED_MIME);
$allowed_file = $valid_extn && $valid_mime;
return $allowed_file;
}
Handling File Upload
Next, create a function handleUpload() and validate the file size, then call the allowedfile() function within it before moving the file to its destination.
function handleUpload() {
$temp = $_FILES['file']['tmp_name'];
$filename = basename($_FILES['file']['name']);
$file_dest = UPLOAD_DIR. $filename;
$is_uploaded = is_uploaded_file($temp);
$valid_size = $_FILES['file']['size'] <= MAXSIZE && $_FILES['file']['size'] >= 0;
if ($is_uploaded && $valid_size && allowedfile($temp, $file_dest)) {
if(move_uploaded_file($temp, $file_dest)) {
$response = 'The file uploaded successfully!';
} else {
$response = 'The file could not be uploaded.';
}
} else {
$response = 'Error: uploaded file size or type is not valid.';
}
return $response;
}
}
File Upload Error Handling
In the same function that we have defined above, write different file error handling cases. PHP returns an appropriate error code along with the file array. It is found in the file error segment or, if an error occurred during the file upload, $_FILES['file']['error'] returns the error code.
// Handle Error
if (!empty($_FILES)) {
echo $error = $_FILES['file']['error'];
switch($error) {
case UPLOAD_ERR_OK:
$response = handleUpload();
break;
case UPLOAD_ERR_INI_SIZE:
$response = 'Error: file size exceeds the allowed.';
break;
case UPLOAD_ERR_PARTIAL:
$response = 'Error: file was only partially uploaded.';
break;
case UPLOAD_ERR_NO_FILE:
$response = 'Error: no file have been uploaded.';
break;
case UPLOAD_ERR_NO_TMP_DIR:
$response = 'Error: missing temp directory.';
break;
case UPLOAD_ERR_CANT_WRITE:
$response = 'Error: Failed to write file';
break;
case UPLOAD_ERR_EXTENSION:
$response = 'Error: A PHP extension stopped the file upload.';
break;
default:
$response = 'An unexpected error occurred; the file could not be uploaded.';
break;
}
} else {
$response = 'Error: Form is not uploaded.';
}
echo $response;
Complete code: PHP File Upload MIME Type Validation with Error Handler
Here, we have merged all the above code, so this is the complete code to upload a file with file size, file mime type validation, and different error handling. This is the complete, secure code for uploading a file using PHP.
<?php
define('UPLOAD_DIR', 'E:/wamp/www/test/form');
define('UPLOAD_DIR', '/var/uploaded_files/');
define('MAXSIZE', 7340032); // allow max 7 MB
$ALLOWED_FILEEXT = array('pdf', 'doc', 'docx' );
$ALLOWED_MIME = array('application/pdf',
'application/msword',
'application/vnd.openxmlformats-officedocument.wordprocessingml.document');
function allowedfile($tempfile, $destpath) {
global $ALLOWED_FILEEXT, $ALLOWED_MIME;
$file_ext = pathinfo($destpath, PATHINFO_EXTENSION);
$file_mime = mime_content_type($tempfile);
$valid_extn = in_array($file_ext, $ALLOWED_FILEEXT);
$valid_mime = in_array($file_mime, $ALLOWED_MIME);
$allowed_file = $valid_extn && $valid_mime;
return $allowed_file;
}
function handleUpload() {
$temp = $_FILES['file']['tmp_name'];
$filename = basename($_FILES['file']['name']);
$file_dest = UPLOAD_DIR. $filename;
$is_uploaded = is_uploaded_file($temp);
$valid_size = $_FILES['file']['size'] <= MAXSIZE && $_FILES['file']['size'] >= 0;
if ($is_uploaded && $valid_size && allowedfile($temp, $file_dest)) {
if(move_uploaded_file($temp, $file_dest)) {
$response = 'The file uploaded successfully!';
} else {
$response = 'The file could not be uploaded.';
}
} else {
$response = 'Error: uploaded file size or type is not valid.';
}
return $response;
}
// Handle Error
if (!empty($_FILES)) {
echo $error = $_FILES['file']['error'];
switch($error) {
case UPLOAD_ERR_OK:
$response = handleUpload();
break;
case UPLOAD_ERR_INI_SIZE:
$response = 'Error: file size exceeds the allowed.';
break;
case UPLOAD_ERR_PARTIAL:
$response = 'Error: file was only partially uploaded.';
break;
case UPLOAD_ERR_NO_FILE:
$response = 'Error: no file have been uploaded.';
break;
case UPLOAD_ERR_NO_TMP_DIR:
$response = 'Error: missing temp directory.';
break;
case UPLOAD_ERR_CANT_WRITE:
$response = 'Error: Failed to write file';
break;
case UPLOAD_ERR_EXTENSION:
$response = 'Error: A PHP extension stopped the file upload.';
break;
default:
$response = 'An unexpected error occurred; the file could not be uploaded.';
break;
}
} else {
$response = 'Error: Form is not uploaded.';
}
echo $response;
?>
<html>
<head>
<title>PHP File Upload MIME Type Validation</title>
</head>
<body>
<form action='#' method="post" enctype="multipart/form-data">
<input type="file" name="file" />
<input type="Submit" value="Submit" />
</form>
</body>
</html>
Related Articles
Complete HTML Form Validation in PHPRemove duplicates from array PHP
PHP remove last character from string
PHP sanitize input for MySQL
PHP secure random password generator
Import a CSV file into MySQL using PHP
PHP copying or moving a file
Create multiple choice questions and answers in PHP
Complete HTML Form Validation in PHP
How to encrypt password in PHP
PHP code to generate Captcha and add in contact form with Validation
How to store Emoji character in MySQL using PHP
PHP File Upload MIME Type Validation with Error Handler
File Upload Validation in PHP
Simple File Upload Script in PHP
jQuery File upload progress bar
Simple star rating system using PHP, jQuery and Ajax
JavaScript display PDF in the browser using Ajax call
jQuery loop over JSON result after AJAX Success